Adding the plugin version to the plugin_row_meta
section in WordPress can be a useful way to display extra information about a plugin directly on the WordPress admin plugins page. This can help developers or site administrators quickly see the plugin version without needing to check the plugin files or visit the plugin settings.
In this guide, we will walk through how to customize the WordPress admin area by adding the plugin version to the plugin_row_meta
. This is particularly useful when managing multiple plugins on your site or when distributing plugins and wanting to make sure users can easily check the current version.
What is plugin_row_meta?
plugin_row_meta
is a WordPress filter that allows developers to modify the meta information shown in the plugins list table on the WordPress dashboard. Normally, this area displays links to the plugin’s website, documentation, support, and other relevant resources.
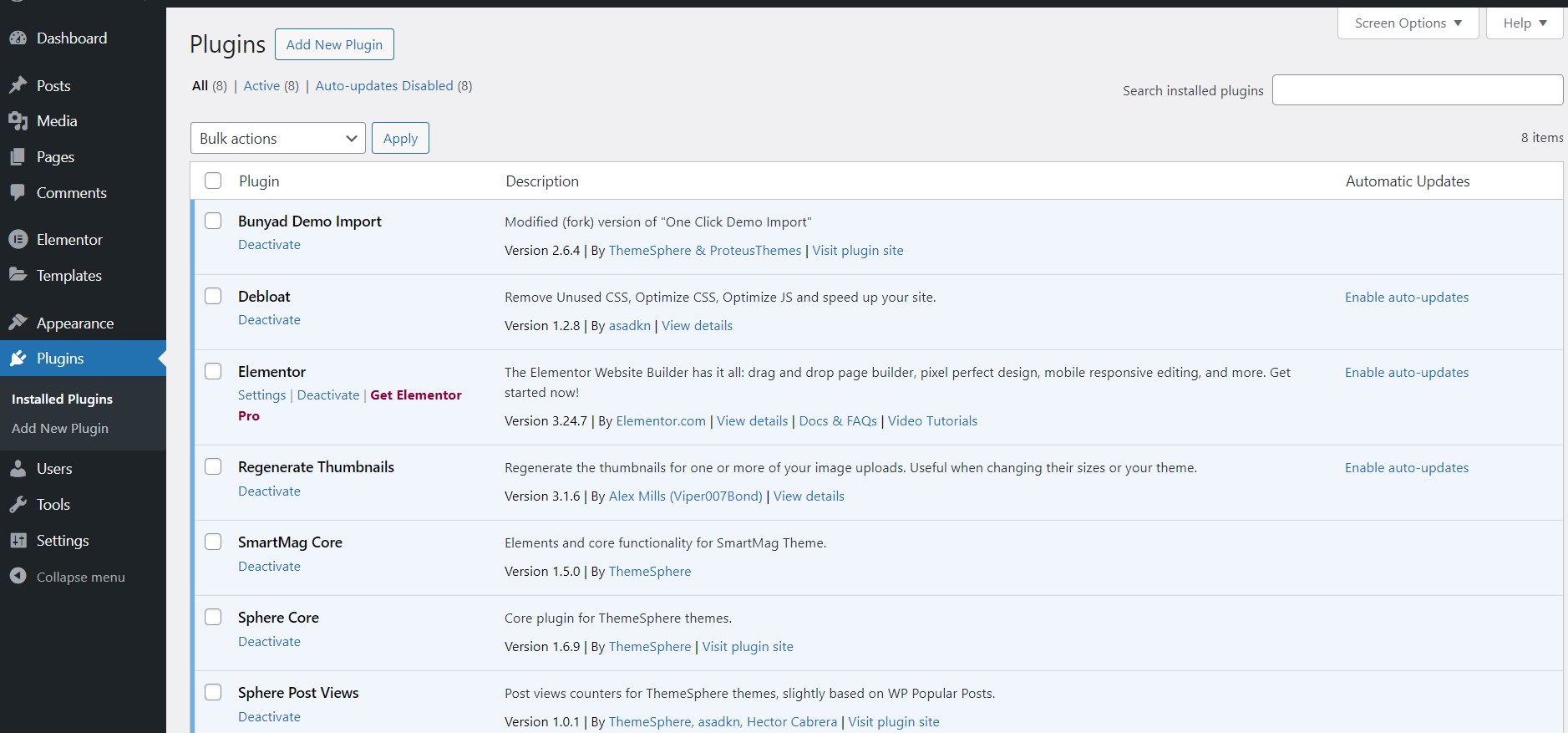
By using this filter, you can add custom links or additional data, such as the plugin version, to the plugin_row_meta
.
Step-by-Step Guide to Add Plugin Version to plugin_row_meta
1. Hook into plugin_row_meta Filter
To add the plugin version to the plugin_row_meta
, we need to hook into the plugin_row_meta
filter. Add the following code snippet to your plugin file or theme’s functions.php
:
add_filter( ‘plugin_row_meta’, ‘add_plugin_version_to_row_meta’, 10, 2 );
function add_plugin_version_to_row_meta( $plugin_meta, $plugin_file ) {
// Specify your plugin file
if ( $plugin_file == ‘your-plugin-folder/your-plugin-file.php’ ) {
$plugin_data = get_plugin_data( WP_PLUGIN_DIR . ‘/’ . $plugin_file );
$version = isset( $plugin_data[‘Version’] ) ? $plugin_data[‘Version’] : ”;
if ( $version ) {
$plugin_meta[] = ‘<strong>’ . esc_html__( ‘Version: ‘, ‘text-domain’ ) . $version . ‘</strong>’;
}
}
return $plugin_meta;
}
2. Explanation of the Code
add_filter( 'plugin_row_meta', 'add_plugin_version_to_row_meta', 10, 2 )
: This function hooks into theplugin_row_meta
filter. We pass the custom functionadd_plugin_version_to_row_meta
which will modify the plugin’s metadata.if ( $plugin_file == 'your-plugin-folder/your-plugin-file.php' )
: This condition ensures that the code only runs for the specific plugin you want to modify. Replace'your-plugin-folder/your-plugin-file.php'
with the actual file path of your plugin.get_plugin_data()
: This function retrieves the plugin header data, including the version number, from the plugin file.$plugin_meta[]
: Adds the plugin version to the$plugin_meta
array, which is then displayed in the plugin’s row meta section.
3. Save and Test
Once you’ve added the code, save the file and navigate to the Plugins page in the WordPress dashboard. You should now see the plugin version displayed in the meta information beneath the plugin name.
Why Add Plugin Version to plugin_row_meta?
There are several reasons why adding the plugin version to plugin_row_meta
can be beneficial:
Quick Version Reference: For developers and site managers maintaining multiple plugins, being able to quickly view the current version of a plugin from the admin interface helps manage updates more efficiently.
Improved User Experience: When distributing a plugin, users can see the version directly on the Plugins page, adding transparency and trustworthiness to the plugin.
Easier Debugging: If you’re troubleshooting plugin conflicts or issues, knowing the plugin version without needing to open the plugin files can save time.
Extending Functionality: Adding More Meta Information
You can go beyond just adding the plugin version and include other useful metadata. For example, you might want to include the plugin’s last update date, author, or a changelog link.
Here’s how you can modify the code to include more data:
add_filter( ‘plugin_row_meta’, ‘add_custom_plugin_meta’, 10, 2 );
function add_custom_plugin_meta( $plugin_meta, $plugin_file ) {
if ( $plugin_file == ‘your-plugin-folder/your-plugin-file.php’ ) {
$plugin_data = get_plugin_data( WP_PLUGIN_DIR . ‘/’ . $plugin_file );
$version = isset( $plugin_data[‘Version’] ) ? $plugin_data[‘Version’] : ”;
$author = isset( $plugin_data[‘Author’] ) ? $plugin_data[‘Author’] : ”;
$last_updated = ‘Last updated on ‘ . date(‘F j, Y’, filemtime( WP_PLUGIN_DIR . ‘/’ . $plugin_file ) );if ( $version ) {
$plugin_meta[] = ‘<strong>’ . esc_html__( ‘Version: ‘, ‘text-domain’ ) . $version . ‘</strong>’;
}if ( $author ) {
$plugin_meta[] = esc_html__( ‘Author: ‘, ‘text-domain’ ) . $author;
}$plugin_meta[] = $last_updated;
}
return $plugin_meta;
}
This will display additional information like the plugin author and the date when the plugin was last modified.